Unlike the incorporation of previous menus, this addition will take place exclusively in the main activity.
We start by defining the menu items in a resource layout XML file as follows:
user@River:~/HolaAndroid$ cat -n res/layout/iconmenu.xml
1 <?xml version="1.0" encoding="utf-8"?>
2 <menu xmlns:android="http://schemas.android.com/apk/res/android">
3 <item android:id="@+id/config"
4 android:title="Config" />
5 <item android:id="@+id/quit"
6 android:title="Quit" />
7 </menu>
8
user@River:~/HolaAndroid$
Next, we modify our HolaAndroid.java source to include the rendering of the menu upon pressing the menu button. We're making use of the Toast utilities to demonstrate the button press. Specifically, lines 13-14 and 57-99 were introduced to get our desired behavior.
user@River:~/HolaAndroid$ cat -n src/com/example/holaandroid/HolaAndroid.java
1 package com.example.holaandroid;
2
3 import android.app.Activity;
4 import android.os.Bundle;
5 import android.widget.Button;
6 import android.view.View;
7 import android.view.View.OnClickListener;
8 import android.util.Log;
9 import android.content.Intent;
10 import android.view.Menu;
11 import android.view.MenuItem;
12 import android.view.MenuInflater;
13 import android.widget.Toast;
14 import android.view.Gravity;
15
16
17 public class HolaAndroid extends Activity
18 {
19 private static final String ClassName = "HolaAndroid";
20 private Button b1_;
21 private Button b2_;
22
23 /** Called when the activity is first created. */
24 @Override
25 public void onCreate(Bundle savedInstanceState)
26 {
27 final String MethodName = "onStart";
28
29 super.onCreate(savedInstanceState);
30 setContentView(R.layout.main);
31
32 b1_ = (Button)this.findViewById(R.id.button1);
33 b1_.setOnClickListener(new OnClickListener() {
34 public void onClick (View v){
35 Log.d(ClassName+"::"+MethodName, "entry");
36 finish();
37 }
38 });
39 b2_ = (Button)this.findViewById(R.id.button2);
40 b2_.setOnClickListener(new OnClickListener() {
41 public void onClick (View v){
42 Log.d(ClassName+"::"+MethodName, "entry");
43 createMenu2();
44 Log.d(ClassName+"::"+MethodName, "entry");
45 }
46 });
47 }
48 private static final int ACTIVITY_CREATE=0;
49 private void createMenu2() {
50 final String MethodName = "createMenu2";
51 Log.d(ClassName+"::"+MethodName, "entry");
52 Intent i = new Intent(this, Menu2.class);
53 startActivityForResult(i, ACTIVITY_CREATE);
54 Log.d(ClassName+"::"+MethodName, "exit");
55 }
56
57 @Override
58 public boolean onCreateOptionsMenu(Menu menu)
59 {
60 final String MethodName = "onCreateOptionsMenu";
61 Log.d(ClassName+"::"+MethodName, "entry");
62 MenuInflater inflater = getMenuInflater();
63 inflater.inflate(R.layout.iconmenu, menu);
64 Log.d(ClassName+"::"+MethodName, "exit");
65 return true;
66 }
67
68 @Override
69 public boolean onOptionsItemSelected(MenuItem item)
70 {
71 final String MethodName = "onOptionsItemSelected";
72 Log.d(ClassName+"::"+MethodName, "entry");
73 boolean retVal=false;
74 switch (item.getItemId()) {
75 case R.id.config:
76 retVal=true;
77 showMsg("Config Select");
78 break;
79 case R.id.quit:
80 retVal=true;
81 showMsg("Quit Select");
82 finish();
83 break;
84 default:
85 retVal=super.onOptionsItemSelected(item);
86 }
87 Log.d(ClassName+"::"+MethodName, "exit");
88 return retVal;
89 }
90
91 private void showMsg(String msg) {
92 final String MethodName = "showMsg";
93 Log.d(ClassName+"::"+MethodName, "entry");
94 Toast toast = Toast.makeText(this, msg, Toast.LENGTH_LONG);
95 toast.setGravity(Gravity.CENTER, toast.getXOffset() / 2, toast.getYOffset() / 2);
96 toast.show();
97 Log.d(ClassName+"::"+MethodName, "exit");
98 }
99
100 }
user@River:~/HolaAndroid$
Make it, and run it and you'll get the following result.
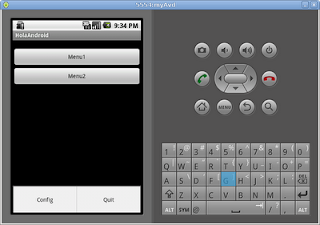
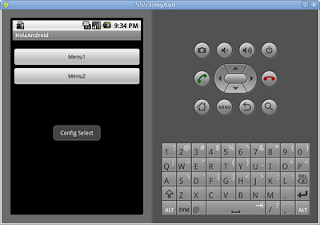